You know what they say... there are 2 hard things in computer science: cache invalidation, naming things and off-by-1 errors.
That's true for feature flags as well. You don't always get the name quite right before the feature flag gets used in production.
But once the flag is in use and tied to your code, how can you change the name without causing issues?
Background
I recently needed to rename a flag, so I thought I'd write up the process for others.
Sure, Cloud makes it easy to rename. Just view the feature, click Edit, change the key and hit Update Feature.
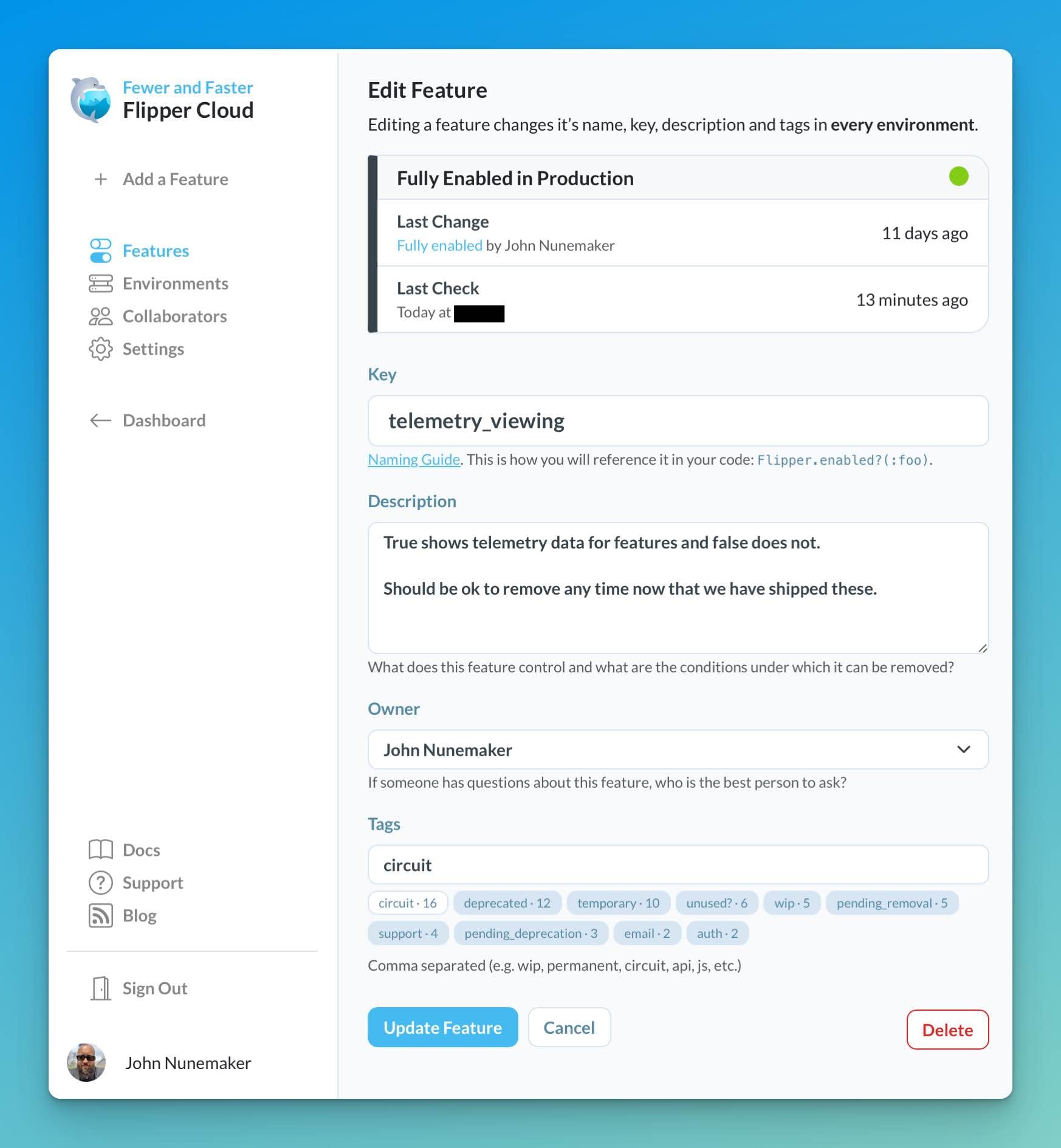
But if you do that without some preparation, your code is still referencing the old key and that's no good.
Why is it no good? Well, renaming the flag like this effectively disables the feature everywhere in your code base immediately (it's the same as the key being missing). If your flag is currently disabled, that's fine. But if it is enabled, that could cause confusion for customers ("hey, where are my analytics!").
The name of the flag I wanted to change was feature_stats
. Since creating and using this flag, I've renamed most of the code to either Analytics
or Telemetry
.
The flag that protects receiving telemetry data is named telemetry_receiving
. Similarly, it made sense to me to rename the feature_stats
flag to telemetry_viewing
. Having both start with telemetry_
just feels more symmetrical.
Chokepoint
Thankfully, I already had an Analytics
module with a ui_enabled?
method that looked like this:
def ui_enabled?(...)
Flipper.enabled?(:feature_stats, ...)
end
Original Analytics.ui_enabled? method.
Because this was used everywhere instead of Flipper.enabled?
directly, I had an easy chokepoint to make the change.
Duplicate
The first thing I did was add a duplicate check with the new flag name:
def ui_enabled?(...)
Flipper.enabled?(:feature_stats, ...) ||
Flipper.enabled?(:telemetry_viewing, ...)
end
Intermediate Analytics.ui_enabled? method.
I deployed the code change, so now the code was checking both flags.
Once the deploy was out, I hopped into Flipper Cloud and renamed the feature from feature_stats
to telemetry_viewing
.
At this point, the old flag feature_stats
was gone and telemetry_viewing
now existed (with retention of audit history and gate values).
Best of all, every customer was seeing their analytics throughout this whole process. There was no point, even for a bit where analytics were disabled.
Cleanup
All that was left at this point was to cleanup after myself. I hopped back into the code and removed the check using the old name like so:
def ui_enabled?(...)
Flipper.enabled?(:telemetry_viewing, ...)
end
New Analytics.ui_enabled? method.
Once that was deployed, I was good to go.
All checks now use the new name and the flag key was changed in Flipper Cloud (while retaining history and values).
Again, this is nothing fancy. But I thought it was interesting that I've been using flags for over a decade and haven't needed to rename one that was in use.
Hopefully this helps you out someday, if you wind up in the same situation.